Build a Sample App
This section guides you through the step-by-step procedure to:
Create an app called Hello World.
Create an action in the app to return Hello World.
Create an action in the app to receive input from the user and return the same as the output.
Before you Start
To build an app, ensure the following aspects:
You must have access to Orchestrate 3.x instance.
You must have a basic understanding of Python (3.6 or later) classes, functions, packages, and data structures.
You will need a PythonIDE to view the app on your local machine. While we recommend using Python IDE, apps can also be written using text editors such as Sublime Text or Notepad++.
Recommended: VS code or PyCharm
Identify the use case for the app to be built.
Understand the App Interface
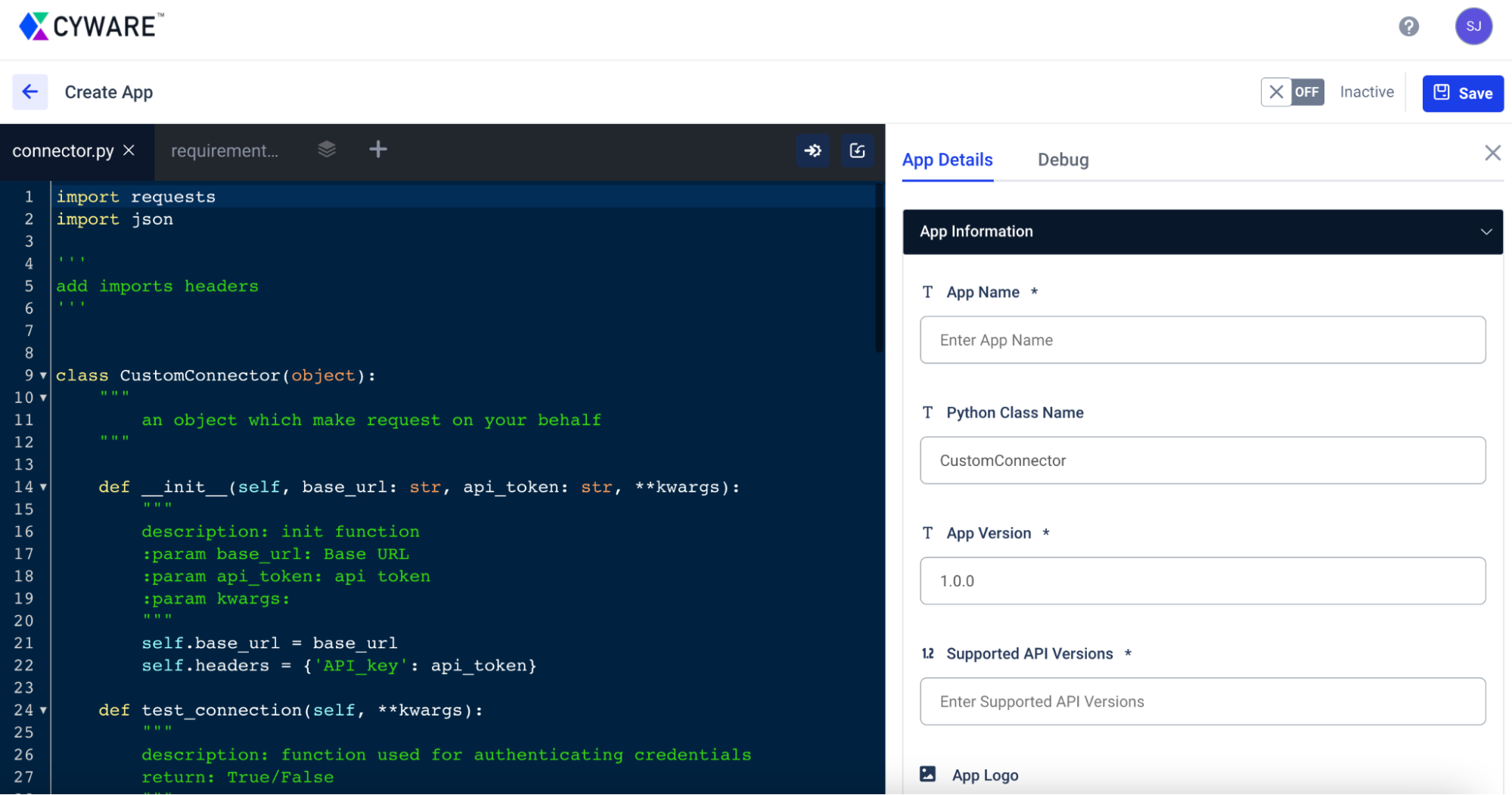
Familiarize yourself with the following components before you start building your first app.
Python-based Interactive Development Environment (IDE) on the left pane: Write your code, and define classes and methods based on the use case of the app you need to build.
App Details on the right pane: Define the app overview, and review the actions and other configurations.
Note
It is important that you sync the data between the two panes whenever you create or make changes to the app. Click the sync icon on the top right of the editor to perform a sync operation from the left pane to the right pane. You cannot perform a sync operation from the right pane to the left.
Understand the usage of the following files:
connector.py file: This is a predefined template, which indirectly serves as your main.py file that executes.
requirements.txt file: Define all the advanced functionalities or any third-party libraries that you want to use. All you have to do is list the libraries and their versions here, and we will install them for you.
_init_.py: The __init__.py file lets the Python interpreter know that a directory contains the code for a Python module. Since all apps are treated as Python modules, the _init_.py file is essential while creating a connector.
Important
Do not delete the _init_.py file and connector.py file.
Steps to Build an App
Follow the steps in this procedure to build a simple “Hello World” app. In Orchestrate, click the Main Menu on the left sidebar and select Apps. In the Apps landing page, click Create New App on the top right of the screen.
Step 1: Create a HelloWorld app with two actis
To create the HelloWorld app, update the connector.py file with the following changes:
Define your class name. Enter your app name followed by the term “Connector”. Make sure you follow this format. For example, HelloWorldConnector
Note
Use the name and capitalization consistently across the connector.py file. Capitals are used to differentiate between the words in the app builder.
Pass the _init_ method. Remove all the parameters and pass this method as follows:
Note
Do not delete **Kwargs while editing the code in the connector.py file
def __init__(self, **kwargs): """ description: init function """ pass
Pass the test_connection method. Replace the authentication code lines with
return True
as shown below, as we are building a simple “HelloWorld” app without authentication. In general, you can use this method to define the authentication methods to connect to the endpoint of the app.def test_connection(self, **kwargs): """ description: function used for authenticating credentials return: True/False """ return True
Remove the auth method, as we are not providing any authentication logic for our “HelloWorld” app.
Remove the request_handler method as we are not making any request calls.
Define the first action that you want the app to perform. In our case, we want to return “Hello World”, so this does not require any input parameters.
Provide a name for your action in the following format: Example: action_hello_world
Note
All methods that follow the format “action_” are considered as actions to the app. If the methods do not have the format mentioned above, then these actions will not be visible in the App UI.
The HelloWorld app does not require any input parameters. Specify the values that must be returned as follows:
def action_hello_world(self, **kwargs): return {"result": "Hello World", "execution_status": "SUCCESS"}
Define other parameters as required. In this case, our next action is to take an input from the user and return the input.
Provide a name for your action in this format: Example: action_return_input
Define one input parameter that accepts a string-based user input and returns the same input as follows:
def action_return_input(self, user_input: str, **kwargs): return {"result": user_input, "execution_status": "SUCCESS"}
Step 2: Synchronize Data
Click the icon on the upper-right corner of the Python IDE to synchronize the data between the IDE and the App Details pane.
Step 3: Configure App Details
In the App Details > App Information section, enter the following details:
Enter a relevant App name. For example, Hello World
Enter the App Version. For example, 1.0.0, since this is the first version of the app.
As we are not using any API calls for the basic “Hello World” connector, enter NA for the API version.
Enter an app description that will be useful to understand the use case of this app. For example, This is a test app and returns Hello World and user input.
Choose a relevant category for the app. For example, IT Services.
Provide a link to the documentation for this app, if it exists.
Skip the App Configuration Parameters section, as we do not need any authentication methods in our example.
In the App Details > Actions section, click on each action to provide a description for reference and verify all actions. You can also provide a description for each parameter if it exists, and click Update. Important: If the action requires an input parameter to execute, then mark that parameter as Mandatory. For the HelloWorld app, the parameter
user_input
for the second actionaction_return_input
will be mandatory.Click Save. The app gets listed on the My Apps page.
Search for the app you have just created. Example: HelloWorld
Step 4: Create a New Instance
Create or define an instance to execute the actions of the app. To do this, launch the app you just created and select the Instances tab.
Click the plus icon to create a new instance icon for the selected version.
Provide an instance name and an expiration date, and click Create.
Step 5: Run App
After you have created the app and specified an instance, you can now run the app.
Click the ellipses on the top right of the page, and select Edit App.
Click the Run icon on the IDE to execute each action before you use this app in a Playbook.
To test an action, select the instance and the action name, and click Test.
Note
It is important you test each action of the app and ensure that the status is displayed as SUCCESS.
If all the actions are successfully executed, you can turn on the toggle status of the app to Active on the top right of the page, and use this app in a Playbook.